Dotnet could not execute because the application was not found or a compatible .NET SDK is not installed
I ran into this interesting issue where my System PATH environment variables got out of order. I ran "dotnet --version" and saw an error I'd not seen before. Dotnet "Could not execute because the application was not found or a compatible .NET SDK is not installed." What's that?
How did I diagnose this?
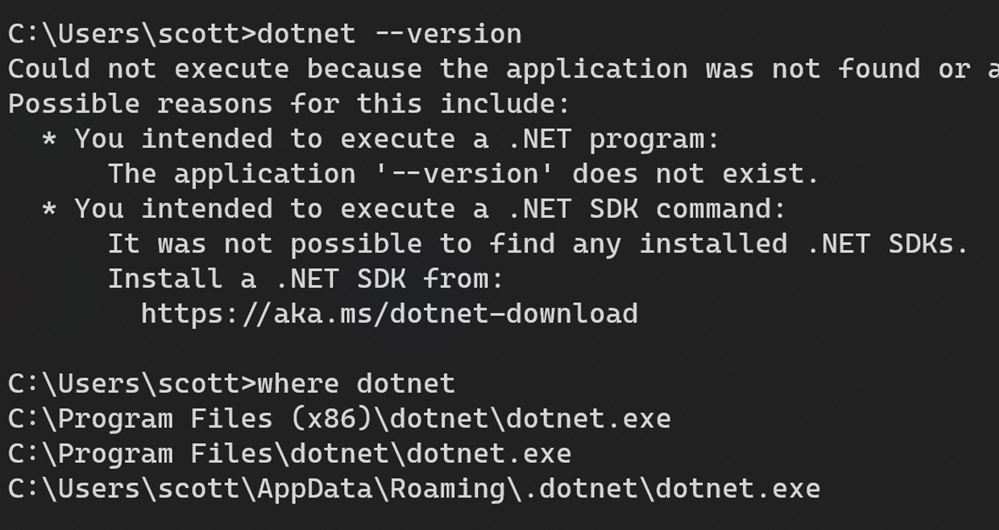
From the command prompt, I typed "where dotnet" to ask cmd.exe "which dotnet.exe are you offering me and in what order?" You would use the which command in Linux, the where command in DOS, and the more explicit where.exe dotnet on PowerShell.
Here I can see that Program Files (x86) has a dotnet.exe that is FIRST in the Path before the x64 version I expected.
Digging into GitHub I can see that the bug has been fixed but it's good to know how things get into a weird state and how easy it is to fix. In this case, I just swapped (or removed) the x86 and x64 (native architecture) paths in my System PATH via the environment variables UI in Windows. Just type Start and Environment Variables, click it, and then Double Click (or Edit) the PATH variable for a nice UI experience. You can even "move up and move down" within that UI - far nicer than editing a text file.
This can happen when you install a 32-bit .NET SDK on a 64-bit system and the last one in wins. In my case, I don't need a 32-bit host at all in my PATH so I ended up just removing it from my PATH completely.
Hope this helps you if you hit it and glad it's fixed.
Sponsor: YugabyteDB is a distributed SQL database designed for resilience and scale. It is 100% open source, PostgreSQL-compatible, enterprise-grade, and runs across all clouds. Sign up and get a free t-shirt!
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter