Exploring the Azure IoT Arduino Cloud DevKit
Someone gave me an Azure IoT DevKit, and it was lovely timing as I'm continuing to learn about IoT. As you may know, I've done a number of Arduino and Raspberry Pi projects, and plugged them into various and sundry clouds, including AWS, Azure, as well as higher-level hobbyist systems like AdaFruit IO (which is super fun, BTW. Love them.)
The Azure IoT DevKit is brilliant for a number of reasons, but one of the coolest things is that you don't need a physical one...they have an online simulator! Which is very Inception. You can try out the simulator at https://aka.ms/iot-devkit-simulator. You can literally edit your .ino Arduino files in the browser, connect them to your Azure account, and then deploy them to a virtual DevKit (seen on the right). All the code and how-tos are on GitHub as well.
When you hit Deploy it'll create a Free Azure IoT Hub. Be aware that if you already have a free one you may want to delete it (as you can only have a certain number) or change the template as appropriate. When you're done playing, just delete the entire Resource Group and everything within it will go away.
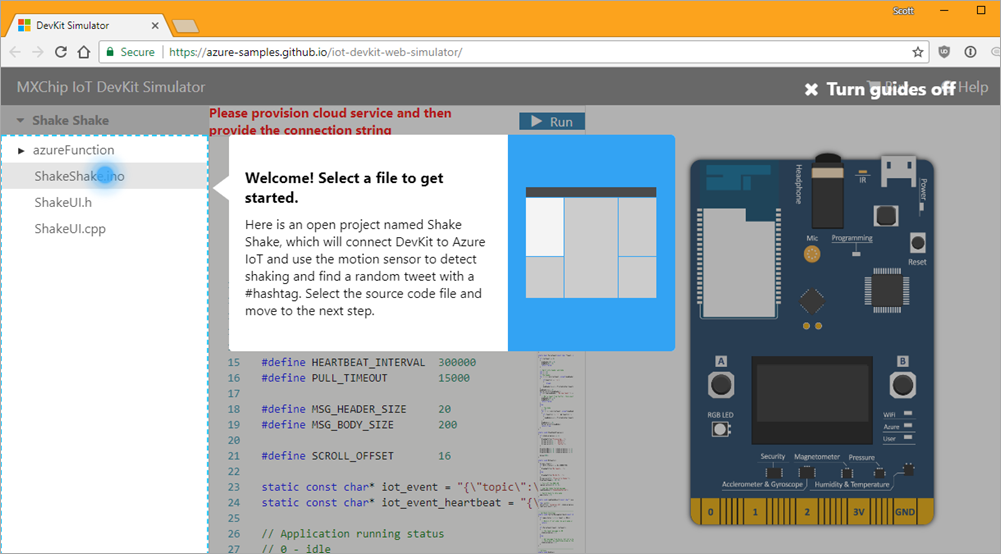
Right off the bat you'll have the code to connect to Azure, get tweets from Twitter, and display them on the tiny screen! (Did I mention there's a tiny screen?) You can also "shake" the virtual IoT kit, and exercise the various sensors. It wouldn't be IoT if it didn't have sensors!
This is just the simulator, but it's exactly like the real MXChip IoT DevKit. (Get one here) They are less than US$50 and include WiFi, Humidity & Temperature, Gyroscope & Accelerometer, Air Pressure, Magnetometer, Microphone, and IrDA, which is ton for a small dev board. It's also got a tiny 128x64 OLED color screen! Finally, the board also can go into AP mode which lets you easily put it online in minutes.
I love these well-designed elegant little devices. It also shows up as an attached disk and it's easy to upgrade the firmware.
You can then dev against the real device with free VS Code if you like. You'll need:
- Node.js and Yarn: Runtime for the setup script and automated tasks.
- Azure CLI 2.0 MSI - Cross-platform command-line experience for managing Azure resources. The MSI contains dependent Python and pip.
- Visual Studio Code (VS Code): Lightweight code editor for DevKit development.
- Visual Studio Code extension for Arduino: Extension that enables Arduino development in Visual Studio Code.
- Arduino IDE: The extension for Arduino relies on this tool.
- DevKit Board Package: Tool chains, libraries, and projects for the DevKit
- ST-Link Utility: Essential tools and drivers.
But this Zip file sets it all up for you on Windows, and head over here for Homebrew/Mac instructions and more details.
I was very impressed with the Arduino extension for VS Code. No disrespect to the Arduino IDE but you'll likely outgrow it quickly. This free add on to VS Code gives you intellisense and integration Arduino Debugging.
Once you have the basics done, you can graduate to the larger list of projects at https://microsoft.github.io/azure-iot-developer-kit/docs/projects/ that include lots of cool stuff to try out like a cloud based Translator, Door Monitor, and Air Traffic Control Simulator.
All in all, I was super impressed with the polish of it all. There's a LOT to learn, to be clear, but this was a very enjoyable weekend of play.
Sponsor: Get the latest JetBrains Rider for debugging third-party .NET code, Smart Step Into, more debugger improvements, C# Interactive, new project wizard, and formatting code in columns.
About Scott
Scott Hanselman is a former professor, former Chief Architect in finance, now speaker, consultant, father, diabetic, and Microsoft employee. He is a failed stand-up comic, a cornrower, and a book author.



About Newsletter